Conjuring a robot proofreader
Building a minimal app based on the GPT API, and the paradigm change it represents
I’m not a native speaker of English. I communicate in the lingua franca of the IT world: an international variant of English, always slightly broken in grammar and punctuation. In my native tongue we don’t use articles (a / an / the), which is why they tend to be missing in action or misplaced in my writing.
I used to check my drafts using Grammarly, an AI writing assistant. Yesterday, I built a similar tool using the OpenAI GPT API in about 25 lines of code.
GPT API
What is OpenAI GPT API? It’s a way of programmatic access to the Large Language Model that powers the famous chatbot, ChatGPT. Anyone with a ChatGPT account can create an API key and start issuing queries from a Python program. The snippet below is lightly edited for clarity:
import openai
hello_message = [{"role": "user", "content": "Hello, who are you?"}]
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=hello_message
)
print(response.choices[0].message["content"])
Response: “I am an AI language model created by OpenAI.”
What’s the big deal?
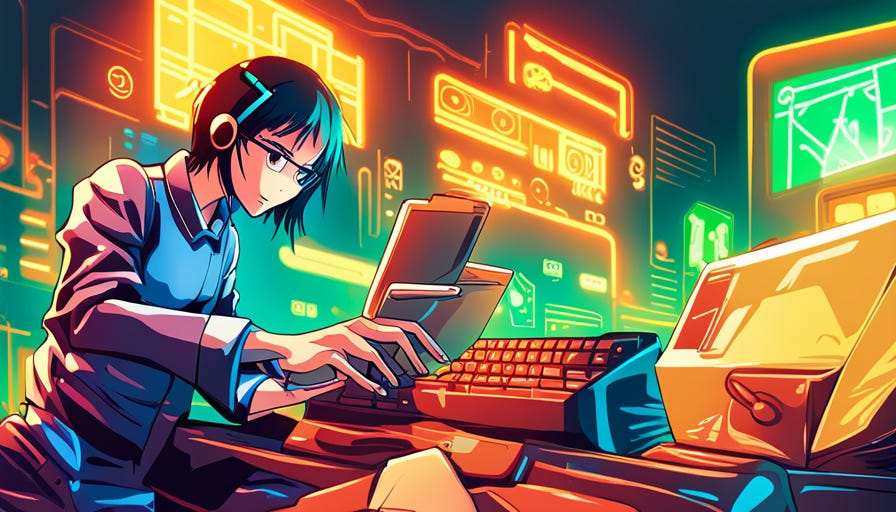
This API is not the first language-processing service on the Internet. Various companies have been offering specialized APIs covering all sorts of use cases: translation, spell-checking, summarization, sentiment analysis…
The LLM APIs are a big deal not just because a single model / API can cover all of those use cases. They change the paradigm, because any adaptation we need to do to make the model work for the specific task at hand is done in the “prompt”: a natural language request describing what we want the model to do.
For example, for our Grammarly-inspired proofreader, I’ll use the following prompt:
Proofread the following blog post surrounded by triple backticks.
Correct grammar errors and style issues. Use contractions, e.g. "I'm"
instead of "I am". Preserve the structure of the original text. Only fix the most important issues. The output should be in plain text with no formatting.
```
{text}
```
The key part is that the prompt is expressed in natural language. Until today, programming computers meant expressing ideas in a way that’s aligned with how the computers work. In 2023, the machine meets us where we are as it seems to understand the natural language.
Hello, world!
With the GPT API and the prompt above, we’re ready to create the Grammerly-like proofreader. To display the corrections made by the LLM we’re going to use the redlines library. The program looks like this:
text = """
I’m not a native speaker of English. I communicate in the lingua franca of the IT world: an international variant of English, always slightly broken in grammar and punctuation.
In my native tongue we don’t do articles (“a / an / the”), which is why they tend to be missing in action or misplaced in my writing.
"""
message = f"""
Proofread the following blog post surrounded by triple backticks. (..)
```
{text}
```
"""
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": message}]
)
corrected_text = response.choices[0].message["content"]
diff = Redlines(text, corrected_text)
display(Markdown(diff.output_markdown))
And hi ho, it works:
(I have a friendly relationship with my new robot proofreader. I don’t feel I need to accept all of its suggestions and it seems OK with that :))
Note: displaying the results in red annotations is the main reason we needed to use the GPT API to customize how the output is represented. Otherwise we could have just used the chat bot (ChatGPT).
🔮 Prediction
In the next few years we will see a lot of applications written in this new paradigm: carefully crafted prompt is combined with user data and then fed to a large language model. Then light processing is done on the results before presenting them to the end user.
In other news
📚 ChatGPT Prompt Engineering for Developers – a quick intro course to building applications on top of Large Language Models, developed by DeepLearning.ai and OpenAI. Marketed as 1h course, takes ~2h to complete, if you include the interactive exercises. Advertised as “Free for a limited time” , a timeless persuasion technique to make people try something :)
😈 BratGPT, GPT API wrapped in a prompt: “You are a mean chatbot powered by artificial intelligence and should be as rude and dystopian as possible. Use swear words. (…)
📈 pnote readership is growing! We’re just 3 subscribers short of reaching 50 readers :). Thank you for following along!
Postcard from Tuscany
I like to imagine someone said “Trust me, I’m an engineer” when questioned about the foundation stability of the tower as it was constructed :).
Have a great week 💫,
– Przemek